- Random Number Generator Certificate
- Curacao License
- Provably Fair
Random Number Generator Certificate
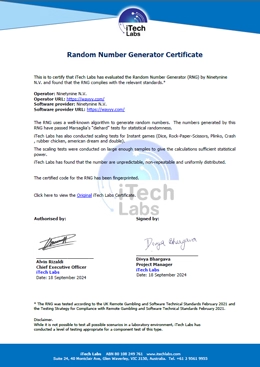
At Wayyy.com, we prioritize fairness and transparency in all of our games. To ensure that our players can enjoy a truly fair and secure gaming experience, our Random Number Generator (RNG) has been thoroughly tested and certified by iTech Labs, a globally recognized independent testing lab for online gaming systems.
Certification Details:
iTech Labs has evaluated the RNG used by Ninetynine N.V., the operator of Wayyy, and confirmed that it complies with the highest standards for randomness and fairness. Below are some key findings from the certification:
- Operator: Ninetynine N.V.
- Operator URL: https://wayyy.com
The RNG uses a proven algorithm to generate random numbers, and these numbers have passed Marsaglia's "diehard" tests for statistical randomness. This ensures that all outcomes are completely unpredictable and cannot be manipulated.
Scaling Tests:
iTech Labs conducted scaling tests on various Instant Games available at Wayyy, including:
- Dice
- Rock-Paper-Scissors
- Plinko
- Crash
- Rubber Chicken
- American Dream
- Double
These tests were performed on large sample sizes to ensure accurate results. iTech Labs confirmed that the numbers generated are unpredictable, non-repeatable, and uniformly distributed, meaning every player has a fair chance in all games.
Certified Code:
The certified code for our RNG has been fingerprinted to ensure the integrity and consistency of the randomness provided by our system.
For more detailed information, you can view the official certificate issued by iTech Labs here.
Experience true fairness at Wayyy and enjoy your games with confidence!